Today I was feeling rather lost in my attempts to understand what is going on inside a Spring Boot application.
Being new to Spring, I had spent a fair bit of time googling and reading, but most of the things i came across simply assume prior knowledge when it comes to fundamental concepts like 'Beans'.
Mark Fisher, my friend and colleague here on the Spring team at Pivotal, came to my rescue and offered to explain. In exchange, I promised to write about it.
The result is this blog post and https://github.com/markfisher/spring-boot-hello-world.
Spring started by providing a much-simpler-than-J2EE, XML-based way to configure the main classes that your Java application depended on.
Simply instantiating everything with new
in Java was not ideal because this meant that you had to hardwire all those dependencies into your code, so you couldn't easily use say one database for local testing and a different database for CI or production.
Instead of writing your own factories which abstracted the creation of things like database connections, you could use Spring and provide the details of all those things in XML.
Spring would read some environment-specific XML at startup and create instances of those classes based on what was in the XML.
And those instances were... Beans, something like this:
Fast forward a few years and the XML has been replaced by Java code annotated as @Configuration
and @ConfigurationProperties
.
@Configuration
@EnableConfigurationProperties(GreeterProperties.class)
public class GreeterAutoConfiguration {
@Bean
public Greeter greeter(GreeterProperties properties) {
return new Greeter(properties.getGreeting());
}
}
Notice that the factory method is annotated as @Bean
.
If the configuration class is included in the META-INF/spring.factories
of a dependent jar, then a Spring Boot application will automatically call the factory method to create a singleton instance of that class on startup.
This is the magic
and those instances are still called Beans
Now that configuration scanning is no longer such a mystery, the role of Spring Boot Starters is easier to understand.
By including a starter in the dependencies of your Boot App, you are telling Spring to scan for configuration classes inside that starter, which results in the automatic creation of the Beans for that starter.
Easy!
šš
{ "path": "/blog/spring-boot-101", "attrs": { "title": "Spring Boot 101", "splash": { "image": "/images/boot1.jpg" }, "date": "2017-06-28", "layout": "BlogPostLayout", "excerpt": "Spring Beans are magic - but what's behind them and why are they called beans?" }, "md": "# Spring Boot 101\n\nToday I was feeling rather lost in my attempts to understand what is going on inside a Spring Boot application.\n\nBeing new to Spring, I had spent a fair bit of time googling and reading, but most of the things i came across simply assume prior knowledge when it comes to fundamental concepts like 'Beans'.\n\nMark Fisher, my friend and colleague here on the Spring team at Pivotal, came to my rescue and offered to explain. In exchange, I promised to write about it.\n\nThe result is this blog post and https://github.com/markfisher/spring-boot-hello-world.\n\n## In the beginning there was XML\n\nSpring started by providing a much-simpler-than-J2EE, XML-based way to configure the main classes that your Java application depended on.\n\nSimply instantiating everything with `new` in Java was not ideal because this meant that you had to hardwire all those dependencies into your code, so you couldn't easily use say one database for local testing and a different database for CI or production. \n\nInstead of writing your own factories which abstracted the creation of things like database connections, you could use Spring and provide the details of all those things in XML.\n\nSpring would read some environment-specific XML at startup and create instances of those classes based on what was in the XML.\n\nAnd those instances were... _**Beans**_, something like this:\n\n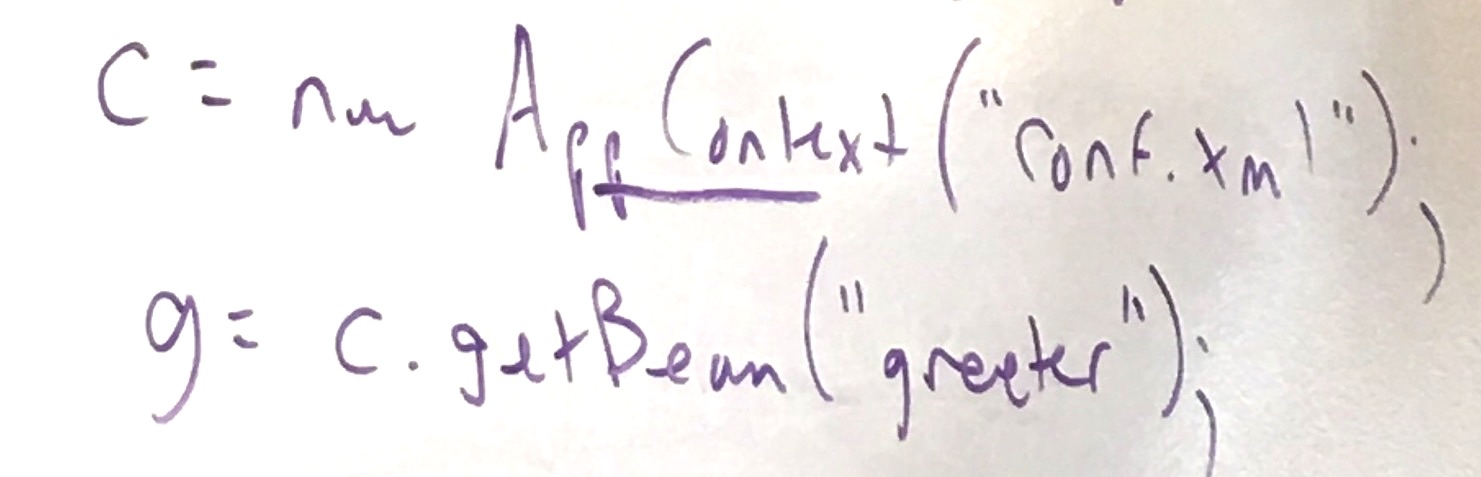\n\n## Now there are @Annotations\n\nFast forward a few years and the XML has been replaced by Java code annotated as `@Configuration` and `@ConfigurationProperties`.\n\n```java\n@Configuration\n@EnableConfigurationProperties(GreeterProperties.class)\npublic class GreeterAutoConfiguration {\n\n\t@Bean\n\tpublic Greeter greeter(GreeterProperties properties) {\n\t\treturn new Greeter(properties.getGreeting());\n\t}\n}\n```\n\n\nNotice that the factory method is annotated as `@Bean`.\n\nIf the configuration class is included in the `META-INF/spring.factories` of a dependent jar, then a Spring Boot application will automatically call the factory method to create a singleton instance of that class on startup.\n\n> This is the magic \n> and those instances are still called **Beans**\n\n## What about [Spring Initializr](https://start.spring.io/) and all those starters?\n\nNow that [_configuration scanning_](https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#using-boot-configuration-classes) is no longer such a mystery, the role of Spring Boot Starters is easier to understand.\n\nBy including a starter in the dependencies of your Boot App, you are telling Spring to scan for configuration classes inside that starter, which results in the automatic creation of the **Beans** for that starter.\n\n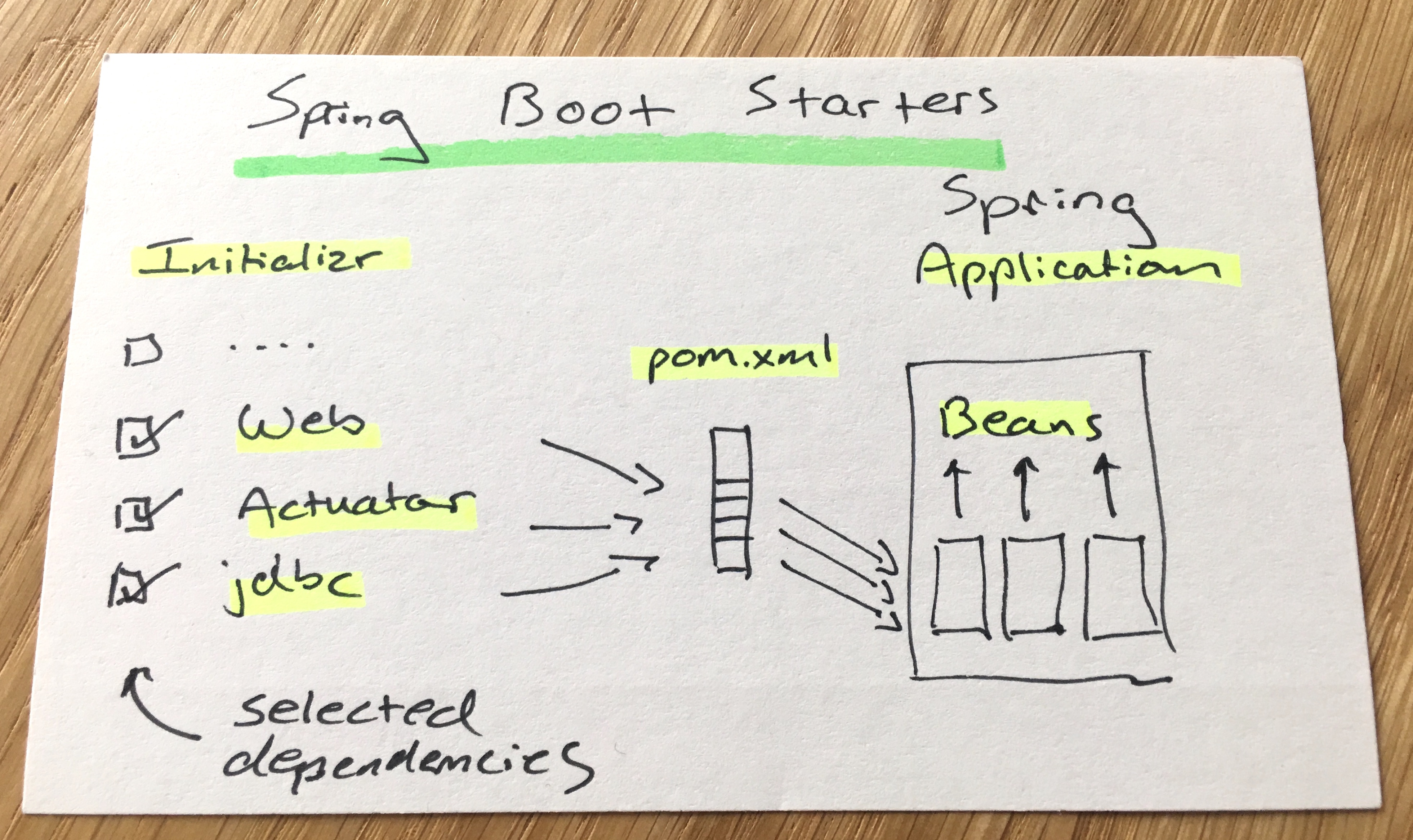\n\n> Easy! \n> šš\n\n---\n", "html": "<h1>Spring Boot 101</h1>\n<p>Today I was feeling rather lost in my attempts to understand what is going on inside a Spring Boot application.</p>\n<p>Being new to Spring, I had spent a fair bit of time googling and reading, but most of the things i came across simply assume prior knowledge when it comes to fundamental concepts like 'Beans'.</p>\n<p>Mark Fisher, my friend and colleague here on the Spring team at Pivotal, came to my rescue and offered to explain. In exchange, I promised to write about it.</p>\n<p>The result is this blog post and <a href=\"https://github.com/markfisher/spring-boot-hello-world\">https://github.com/markfisher/spring-boot-hello-world</a>.</p>\n<h2>In the beginning there was XML</h2>\n<p>Spring started by providing a much-simpler-than-J2EE, XML-based way to configure the main classes that your Java application depended on.</p>\n<p>Simply instantiating everything with <code>new</code> in Java was not ideal because this meant that you had to hardwire all those dependencies into your code, so you couldn't easily use say one database for local testing and a different database for CI or production.</p>\n<p>Instead of writing your own factories which abstracted the creation of things like database connections, you could use Spring and provide the details of all those things in XML.</p>\n<p>Spring would read some environment-specific XML at startup and create instances of those classes based on what was in the XML.</p>\n<p>And those instances were... <em><strong>Beans</strong></em>, something like this:</p>\n<p><img src=\"/images/boot1b.jpg\" alt=\"ApplicationContext.getBean()\"></p>\n<h2>Now there are @Annotations</h2>\n<p>Fast forward a few years and the XML has been replaced by Java code annotated as <code>@Configuration</code> and <code>@ConfigurationProperties</code>.</p>\n<pre><code class=\"language-java\">@Configuration\n@EnableConfigurationProperties(GreeterProperties.class)\npublic class GreeterAutoConfiguration {\n\n\t@Bean\n\tpublic Greeter greeter(GreeterProperties properties) {\n\t\treturn new Greeter(properties.getGreeting());\n\t}\n}\n</code></pre>\n<p>Notice that the factory method is annotated as <code>@Bean</code>.</p>\n<p>If the configuration class is included in the <code>META-INF/spring.factories</code> of a dependent jar, then a Spring Boot application will automatically call the factory method to create a singleton instance of that class on startup.</p>\n<blockquote>\n<p>This is the magic<br>\nand those instances are still called <strong>Beans</strong></p>\n</blockquote>\n<h2>What about <a href=\"https://start.spring.io/\">Spring Initializr</a> and all those starters?</h2>\n<p>Now that <a href=\"https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#using-boot-configuration-classes\"><em>configuration scanning</em></a> is no longer such a mystery, the role of Spring Boot Starters is easier to understand.</p>\n<p>By including a starter in the dependencies of your Boot App, you are telling Spring to scan for configuration classes inside that starter, which results in the automatic creation of the <strong>Beans</strong> for that starter.</p>\n<p><img src=\"/images/boot7.jpg\" alt=\"Initializr injection\"></p>\n<blockquote>\n<p>Easy!<br>\nšš</p>\n</blockquote>\n<hr>\n" }